Create a new component
Creating a custom component from scratch
If the JBStrap framework components are not enough, you can create a new component from scratch. This new component must extend from the Component class.
This component creation process requires much more work from your part, but it does allow you to create components from scratch. In the following example, a component called Rating will be created. The component will allow the user to rate certain page elements on a 5-star scale. This example component isn’t very complex, you can query the score from it, or modify the score. If the rating score is modified, the component will be automatically redrawn on the user interface.
Implementation
Custom components must extend from the Component class. This class contains every method that is needed for the operation of a component. If your component does not extend from this class, your component won’t be able to behave as a component in the application – it cannot be placed, and component settings do not apply to it.
In the above source code, the writerHTML method draws the component to the interface. This is an abstract method from the Component class, as such, every class that extends from the Component class must implement this method. The method has to receive the writer class, that will contain the HTML code that is to be drawn. You can use any HTML content here, as it will be placed where the component is. This HTML code will represent the component on the client-side.
The main HTML tag of the component must be opened with the openTag method. This not only contains the tag, but the component id, the style class applied to the component, along with the attributes to be applied. The main tag can be closed with the closeTag method. Both methods have one parameter, the tag that represents the component in the HTML code. Every other HTML tag can be written freely within the method that draws the component.
If your component has event handling methods assigned to it, then those will be assigned to the component’s main tag in the HTML code, as that tag represents the component.
The method that draws the component must return with a Boolean value, that specifies to the framework, if the component was successfully drawn or not. Thus, in this example, it will return with a constant true value, since the component will be always successfully drawn.
Running the above example will display 5 stars on the page. The first three will be orange, the last two will be dark, representing a 3 star rating. The end result should look like this:
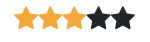