JBStrap File Handling
The JBStrap framework comes with a file handling layer. This layer ensures the saving, reading and deletion of files. File names and contents are stored separately and you can store multiple files that have the same name. Another advantage of the layer is that the paths of the files in the FileStore s do not have to be hard-coded, they can be stored in parameters, thus you can easily move files without modifying the code.
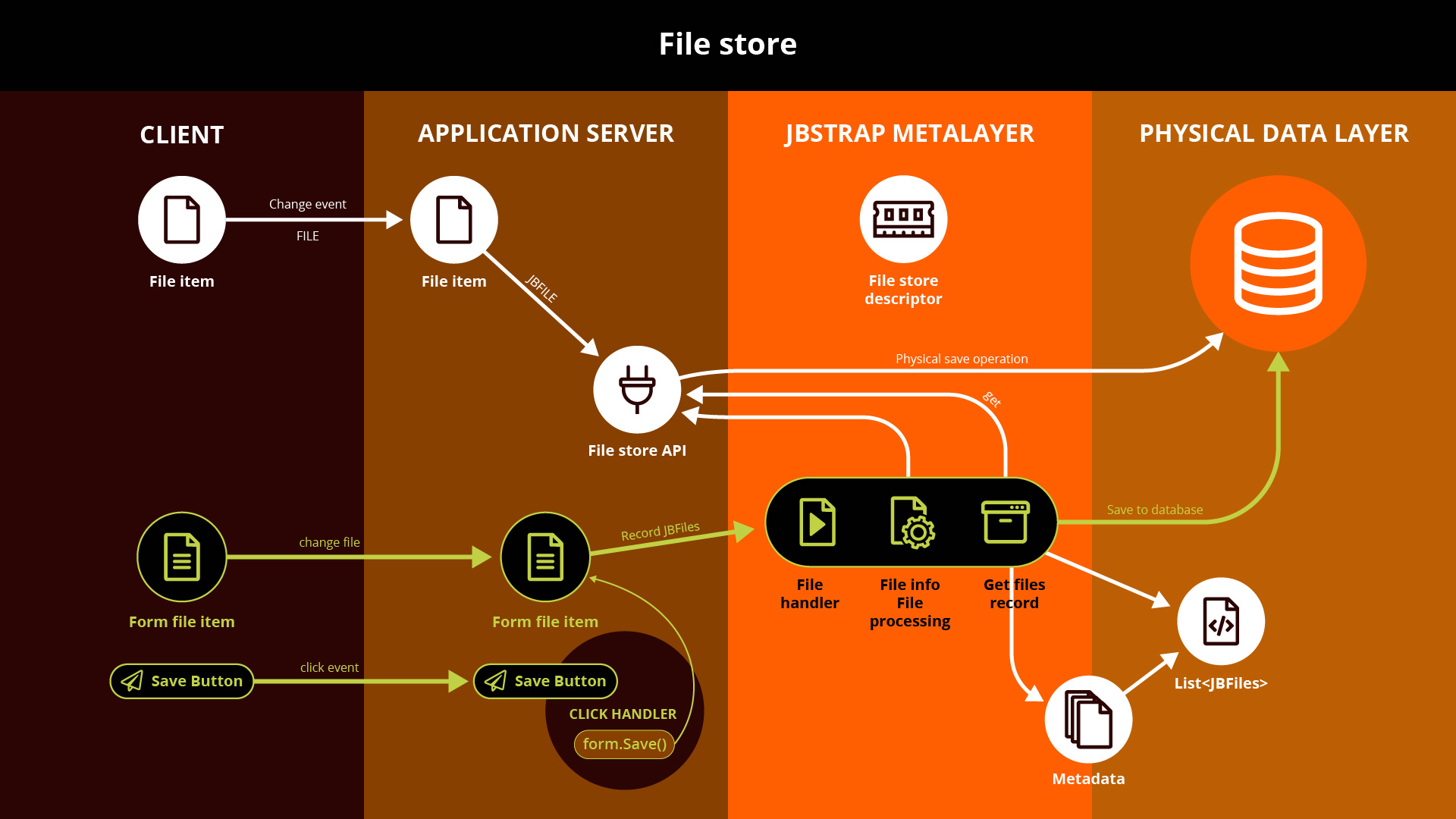
The file storing logic can be specified in the FILE_STORE_BASE JBStrap parameter. You need to specify a basic path, that will be used by every FileStore . This path must be specified as an URL string, meaning that the files could be stored on the server’s own file system, external storage, or using a different network location or different server.
You can create a single, or multiple FileStore s. You can assign their own relative paths. These paths are relative to the ones specified in the FILE_STORE_BASE parameter. The relative paths must be specified in plain text format. Every file stored in the FileStore will be stored in the specified location. The files will receive a unique ID, that will be used as its name in the server file system. To access these files, you have to use these IDs, so the storage of these must be handled by the program logic. If the file was created during the saving of a Record, or if the file is stored with the help of a data handling operation, the storing of the file’s meta data is handled by the file storage method, which was specified for the framework (in this case in the FileHandler implementation). This is detailed in the File Storage section.
JBFile
The files in the framework are represented by the JBFile class. This class provides the storing of the fields and their metadata. These are stored in an object. The class also provides the file’s basic operations. Every component and file handling supports the use of the JBFile class, instead of the basic java File class. You can access this basic File class, through the JBFile class, if you need it. You can create new files, delete a file, or send a file to another client (downloading the file) through this class as well.
Functionalities
You can create a JBFile in multiple ways. Most of the time, you need to create a JBFile, that has its contents previously made. One such case could be generating a file, when the file contents are already done, and you only want to store it in the FileStore, as a file.
You can also create files in any custom way, you only must be able to give its contents, in a byte array, to the JBFile class. For example, you want to place an existing file to the FileStore (copying it), then you have to do the following:
If you created a JBFile previously, or if a component has a JBfile, you can easily store them in the FileStore, by using the store method. The file will be saved to the FileStore, that was specified in the JBFile class.
If you have a JBFile class, which was read from a FileStore, the file can be downloaded by a client, meaning that the user’s browser will start to download the file:
You can also delete a file by using the delete method. The file’s meta data will still be available in the JBFile class, but the physical file itself will be deleted. This cannot be undone.
Using the JBFile class, you can specify the exact file size, in bytes, or in human readable format. This latter means that the file size will be returned with the proper unit, in a textual form, that can be read easily by humans.
- If the file is smaller than 1 kilobyte, the file size will be displayed in bytes: 324 byte
- If it is between 1 kilobyte and 1 megabyte, the file size will be in kilobytes: 34 KByte
- If it is between 1 megabyte and 1 gigabyte, the file size will be in megabytes: 52 MByte
- If it is between 1 gigabyte and 1 terabyte, the file size will be in gigabytes: 76 GByte
- If it is more than one terabyte, it will be in terabytes: 56 TByte
You can also get the file’s contents and metadata from the JBFile class: