JBStrap file storage
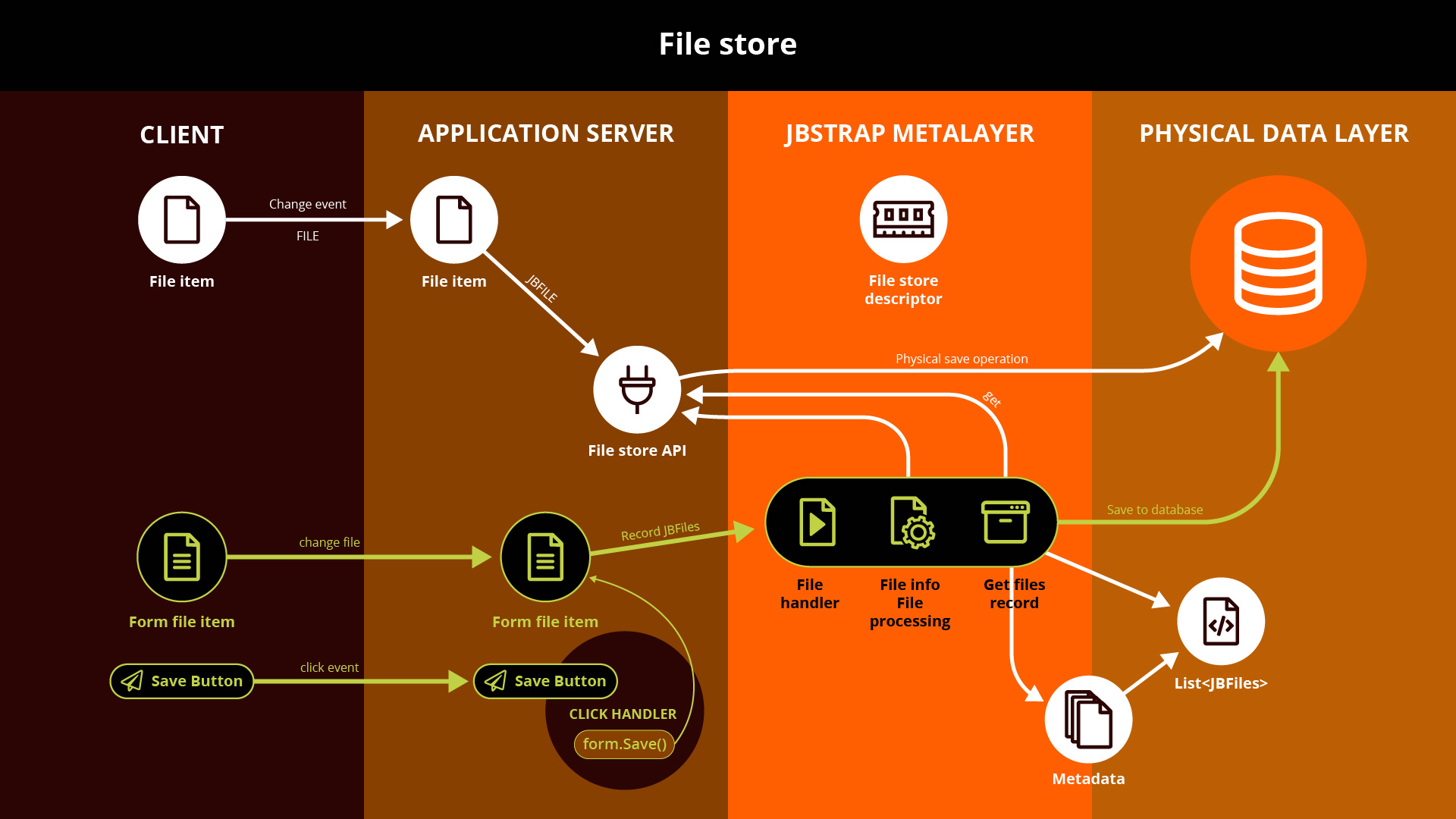
If a data record is saved using a DataDescriptor contains a column whose value is a list of JBFile elements, the file is automatically saved to the corresponding FileStore . If data is retrieved from a DataDescriptor that has a file type field, it will be read automatically and the list returned by the query will contain JBFile objects. This solution is fully compatible with both the FileItem editor component and the Form component. That is, when a DataDescriptor with a file type field is displayed on an editor interface, a file type column will display a FileItem editor component. Here the user can upload a file or multiple files. When you save the Form or associated data records using a DataDescriptor , the uploaded files will automatically be saved to the referenced FileStore . If you open this file for editing, previously uploaded files will be displayed in the FileItem component, which can be downloaded or deleted by the user. If the user deletes a previously uploaded file in this way, the file will be deleted from the file store when saving.
The JBStrap framework provides a general solution for storing files, which will be described in detail in the next section. In addition, we can implement a file storage mechanism, in which case both the form component and data source operations will be performed using the custom file manager.
Default file handler
If you want to use the default file handler provided by the framework, you need to add a text column to the data table where the file is uploaded, in which the file handler stores the metadata for files. The column size should be sufficient for metadata. Thus, a column with a smaller size is sufficient if a single file is to be uploaded, but a column with a larger size is needed if the user is allowed to upload multiple files. Accordingly, the default file handler will store the metadata for uploaded files in each data record, but the physical file will not be saved in a database but the specified file handler and retrieved from there. If the metadata stored by the file handler is corrupted, the file will still be readable by the file system, however, automatic retrieval may not function properly.
Custom file handler
To create your own file manager, you need to create a class that extends the FileHandler class. This class is an abstract class that requires the implementation of three methods. The following methods are used for file management:
processFilesBeforePersist()
This method is called before the data record is saved. The following parameters are passed to the method:
- List<JBFile> : A list of the files that are included in a given data record
- DataRecord : The data record that the user is about to save
- String : The name of the attribute that contains the files in the data record
- Client : The client that initiated save
Using this information, the method must store the file's metadata and save the file to the corresponding FileStore . When you save the file to the FileStore , the file gets a unique identifier, which must also be stored in the metadata of the file, as this is how you will be able to retrieve the file from the FileStore later.
This method is suitable for saving only newly created files, due to stored save transaction history that may have to be rolled back. The operations in the file system do not support the transaction; if you store only new files here, data will be kept unchanged.
If the method returns an error, the save operation is interrupted and program execution is rolled back to the call point.
processFilesAfterPersist()
This method is called after saving the data record. The method receives the following parameters that you can use during processing:
- List<JBFile> : A list of the files that are included in a given data record
- DataRecord : The data record that the user is about to save
- String : The name of the attribute that contains the files in the data record
- Client : The client that initiated the backup
Using this information, the method must perform corresponding operations on files and their associated metadata. At this point, you can delete and modify files, because the data record has been successfully saved in the database.
If the method returns an error, the save operation is interrupted. The state of the data record stored in the database is reverted to its original one and program execution is rolled back to the call point.
getFiles()
This method is called when you retrieve files. The method receives the following parameters, which you must use to restore files:
- DataRecord : A data record retrieved from the database without files
- String : The name of the attribute which represents files in the DataRecord
- Client : The client that initiated retrieval
The method must generate a list based on the received parameters and return a list of type <JBFile> that contains the file or files matching the request. If there is only one file in the data record, the file must be returned in a list.
During processing, the method must determine the metadata of the files based on the parameters, and retrieve the file from the FileStore based on the metadata and the unique identifier of the file. The resulting list must be returned by the method.
If the method throws an exception, the read operation is interrupted and program execution is rolled back to the method call point.